Hello fellow Go enthusiasts, and welcome to the starter's guide to reinforcing your web fortresses! If you've been coding in Go long enough to appreciate its conciseness and efficiency, you'll know that simplicity in Go is like adding extra bacon to your burger – it just makes everything better. But when it comes to web security, cutting corners is like forgetting to cook the bacon — a definite nope!
That's where JWT, or JSON Web Tokens, come into the picture. They're like VIP passes for your users, letting them access the exclusive club of your application's protected routes, all while keeping gatecrashers out. Elegant, secure, and incredibly easy to implement in Go, JWTs are about to become your new best pals.
In this guide, we'll go on a code-filled journey through the implementation of JWT authentication in your Go applications. So gear up with your favorite IDE, and let's start coding our way to a more secure Go-verse!
What are JWT Tokens?
JWT tokens are a compact, URL-safe means of representing claims to be transferred between two parties. Think of claims as little nuggets of information that you can trust because they are digitally signed.
JWT Structure = Header + Payload + Signature
And why, you might ask, do these tokens fit so snugly with our beloved Go? Because just like Go, JWTs are straightforward, efficient, and get the job done without any fuss.
Structure of JWT: Header, Payload, Signature
A JWT typically consists of three parts:
- Header: Specifies the token type and the signing algorithm.
- Payload: Contains the claims (statements about an entity and additional data).
- Signature: Used to verify that the sender of the JWT is who it says it is and to ensure that the message wasn't changed along the way.
{
"alg": "HS256",
"typ": "JWT"
}
This trinity works together to create an encoded string that looks somewhat like gibberish to humans but makes perfect sense to machines. And in the end, isn't that the goal of every developer's creation?
Why JWT is a good fit for Go
Go's standard library already packs quite the punch, but when coupled with JWT, you get a dynamic duo that Batman and Robin would envy. No need for an Alfred to manage all your authentications, because Go and JWT can handle all that heavy lifting with just a few lines of code.
By the end of this guide, you will have written less code than it takes to argue whether tabs or spaces are superior—which, as we all know, is an argument best left untouched in the Go community!
Stay tuned as we take Go and JWT from 'just friends' to 'partners in crime'. Let's begin our tale of `tokens`, one `go run` at a time!
Setting Up the Go Environment
Welcome back, navigators of the byte sea! Let's get our Go environment shipshape for JWT token authentication. We are going to use the github.com/golang-jwt/jwt/v5
package for this quest, as it's the community-trusted guardian when it comes to handling JWT in Go.
Installing Necessary Go Packages
First things first, let's haul in the JWT package. Open your terminal and let the magic of Go Modules do its thing:
$ go get github.com/golang-jwt/jwt/v5
Easy as pie, right? You just Go-Get what you need. Remember, managing dependencies in Go is like a skilled juggler—it's all about keeping your modules in balance.
Configuration Settings for a JWT-Enabled Project
With the package in place, it's time to set sail on the configuration. You don't need a compass to navigate this part, just a good ol' text editor to create a `main.go` file in your project's root directory.
Here's a glimpse of what your boat—err, I mean, starting code—should look like:
package main
import (
"fmt"
"log"
"net/http"
"github.com/golang-jwt/jwt/v5"
)
func main() {
http.HandleFunc("/token", GetTokenHandler)
log.Println("Starting server on :8080...")
log.Fatal(http.ListenAndServe(":8080", nil))
}
// We'll define this handler in the 'Generating Tokens' section, stay tuned!
func GetTokenHandler(w http.ResponseWriter, r *http.Request) {
// Placeholder for token creation logic
fmt.Fprintln(w, "Token creation logic will come here!")
}
Note the inclusion of our jwt package. It's silently waiting for its debut, like the quiet kid in class who turns out to be a genius poet. But don't worry, we'll put it to use soon enough when we create our JWT tokens.
You are now equipped with the JWT package, and your Go environment is primed to build a fortress of tokens. That's all for this chapter, but hold on to your keyboards! Up next, we're crafting our JWT middleware—one step closer to a burglar-free application.
Creating the JWT Authentication Middleware
Alright, codewrights, it’s time to forge the steel gates of our JWT middleware! This nifty function will stand guard, ensuring that only those bearing valid JWTs may pass through to our sacred Go grounds.
We're building this middleware using our trustworthy sidekick, the github.com/golang-jwt/jwt/v5
package. Let’s lock and load!
Writing the JWT Middleware Function
First, let's draft our middleware function. This function will wrap around our handlers, checking for the JWT in each request's Authorization header.
package main
import (
"fmt"
"net/http"
"strings"
"github.com/golang-jwt/jwt/v5"
)
// Our assumed secret for signing tokens
var jwtKey = []byte("my_secret_key")
// JWTMiddleware checks for the validity of the JWT tokens
func JWTMiddleware(next http.Handler) http.Handler {
return http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) {
// We expect the "Authorization" header to be "BEARER {TOKEN}"
authHeaderParts := strings.Split(r.Header.Get("Authorization"), " ")
if len(authHeaderParts) != 2 || authHeaderParts[0] != "Bearer" {
http.Error(w, "Authorization header format must be 'Bearer {token}'", http.StatusUnauthorized)
return
}
// Get the JWT token from header parts
tokenStr := authHeaderParts[1]
// Initialize a new instance of `Claims`
claims := &jwt.RegisteredClaims{}
// Parse the JWT string and store the result in `claims`.
token, err := jwt.ParseWithClaims(tokenStr, claims, func(token *jwt.Token) (interface{}, error) {
return jwtKey, nil
})
if err != nil {
if err == jwt.ErrSignatureInvalid {
http.Error(w, "Invalid token signature", http.StatusUnauthorized)
return
}
http.Error(w, "Bad request", http.StatusBadRequest)
return
}
if !token.Valid {
http.Error(w, "Invalid token", http.StatusUnauthorized)
return
}
// Token is valid, pass it through the next handler
next.ServeHTTP(w, r)
})
}
Bam! Our function takes no prisoners—only valid tokens, please. Invalid requests get a nice 401 "wrong door, buddy!" or a slightly more forgiving 400 if they're just lost.
Validating the Tokens in Requests
The jwt.ParseWithClaims
function swings into action, validating our JWT. Notice how we’re using jwt.RegisteredClaims
. Standard claims, such as expiry time, are directly at your disposal.
Middleware Integration with the Go net/http
Package
Finally, let's hook our middleware into the Go machinery. We simply wrap our existing handlers:
func main() {
// Create a new instance of the mux router
myRouter := http.NewServeMux()
// Our dummy handler for demonstration
myRouter.Handle("/protected", JWTMiddleware(http.HandlerFunc(ProtectedEndpoint)))
log.Println("Server starting on :8080...")
log.Fatal(http.ListenAndServe(":8080", myRouter))
}
func ProtectedEndpoint(w http.ResponseWriter, r *http.Request) {
fmt.Fprintln(w, "You've reached the protected endpoint!")
}
There you go, you've got your own JWT bouncer checking IDs at the door of your "/protected" route. No need for velvet ropes when you have Go and JWT as your entourage!
And what do we have here at the end of this construction? A middleware masterpiece! Next stop, we're off to the token generation station! Keep those engines running, and don't touch that dial.
Generating Tokens
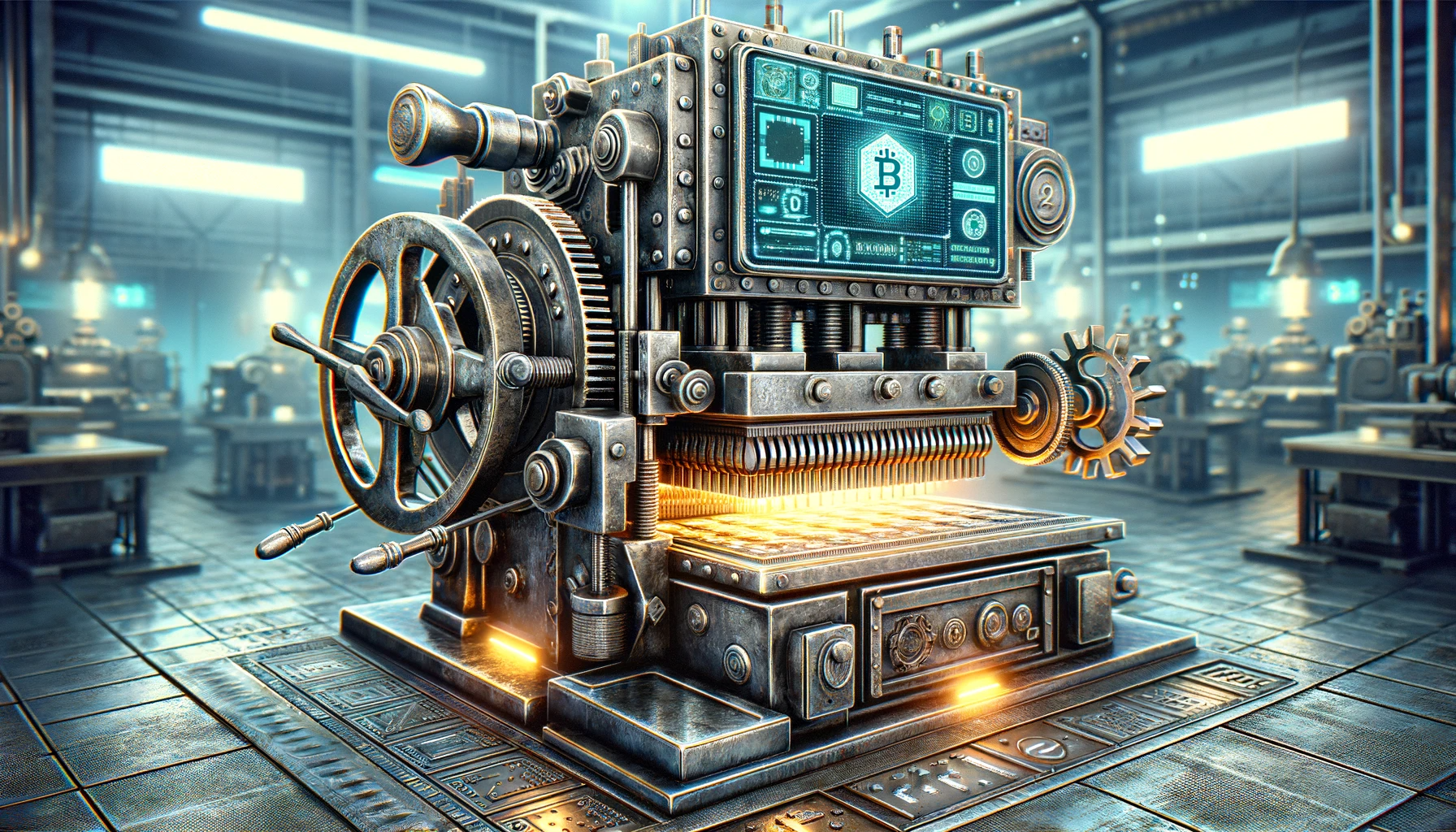
Now for the pièce de résistance, where we play our part as the minters of digital gold – generating JWT tokens. The tokens we create will be the keys to our kingdom, allowing safe passage to those we deem worthy.
Setting up the Route Handler to Issue JWTs
Let’s roll up our sleeves and outline the high-stakes blueprint for the route which will bequeath our treasured tokens.
package main
import (
"time"
"net/http"
"github.com/golang-jwt/jwt/v5"
)
const JwtKey = "YourSuperSecretKey"
func TokenGenerator(w http.ResponseWriter, r *http.Request) {
expirationTime := time.Now().Add(5 * time.Minute)
claims := &jwt.RegisteredClaims{
ExpiresAt: jwt.NewNumericDate(expirationTime),
Issuer: "YourAppName",
}
token := jwt.NewWithClaims(jwt.SigningMethodHS256, claims)
tokenString, err := token.SignedString([]byte(JwtKey))
if err != nil {
w.WriteHeader(http.StatusInternalServerError)
return
}
w.Write([]byte(tokenString))
}
func main() {
http.HandleFunc("/generate", TokenGenerator)
// ... other configurations
}
Within this secret-spawning sanctuary, we designate an expiration for our tokens, engrave our claims, and select the reliable HS256 algorithm as our trusty steed.
Defining Claims and Signing the Token
We’ve sketched out the expiration date like an expiration on a carton of milk – don't let it go sour. We also define the issuer, as any good storyteller would, marking the origin of our tale.
token := jwt.NewWithClaims(jwt.SigningMethodHS256, claims)
tokenString, err := token.SignedString([]byte(JwtKey))
if err != nil {
// Handle the error, perhaps with an HTTP 500 status code
}
Then, with a flick of our cryptographic wand, we sign our tokens with the elegance of a fabled calligrapher. Alas! Our tokens are ready to be dispatched to the masses.
Refresh Tokens and Their Management
Like any good fortress, we also have our secret passages, or “refresh tokens.” These allow the bearer to gain a new token, should the old one meet its inevitable demise (expiration). The management of such tokens may lead to new quest lines, which – due to our current scroll length – shall be reserved for future sagas.
Token generation is complete. Our users can now authenticate with the might of our secured endpoints. Forward, guardians of code, to the wondrous realms of validation and utilization!
Securing Endpoints with JWT
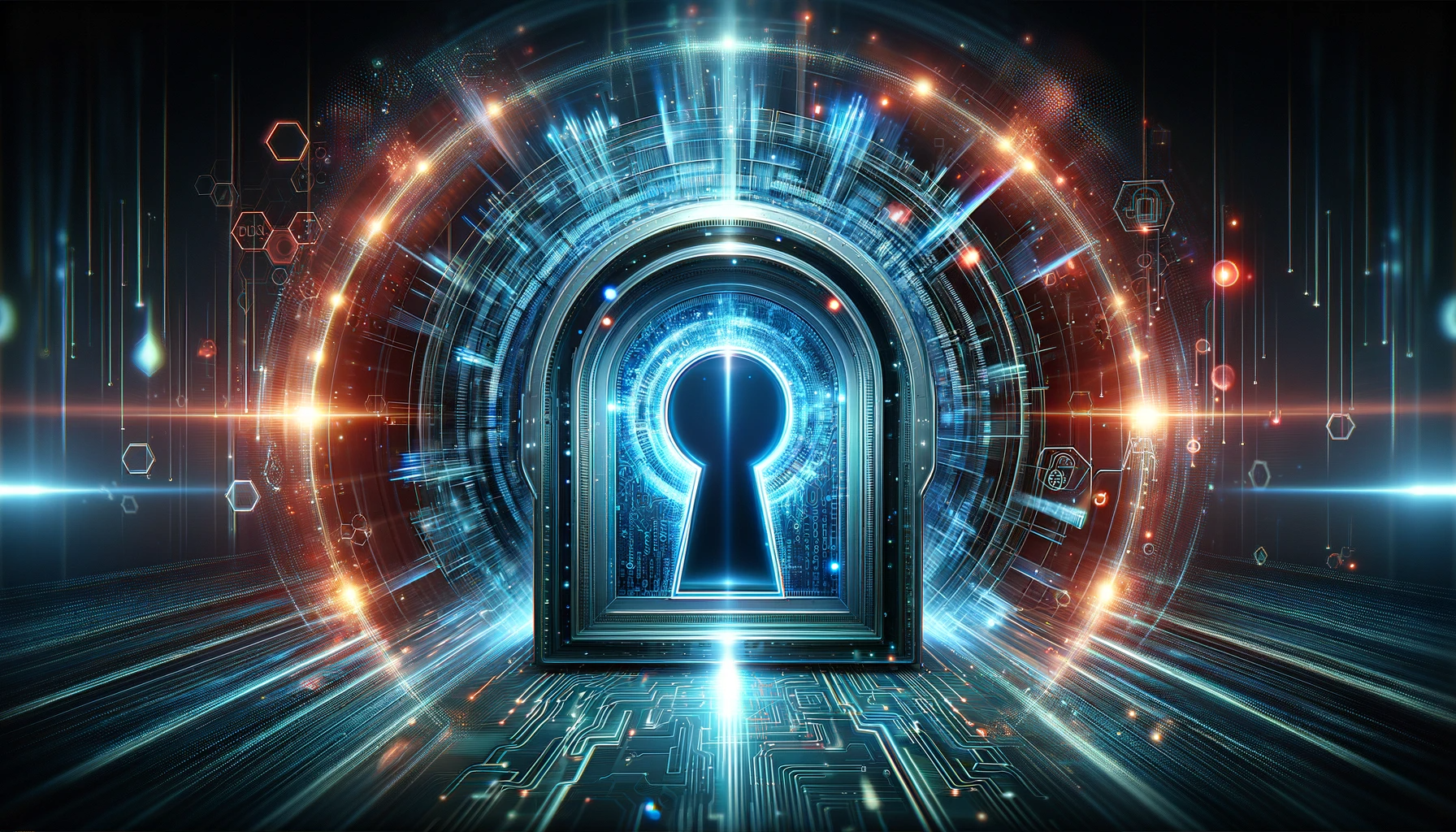
Gather 'round fellow developers, as we fortify our endpoints in Go like master castle architects. With our trusty JWT in hand, we'll seal the gates from marauding requests seeking entry without the proper passphrase.
Applying the JWT Middleware to Protect Routes
Let's weave in our JWT middleware like a well-placed spell of protection. It's the incantation that will whisper "You shall not pass" to unauthorized requests aiming to breach our secured endpoints.
package main
import (
"fmt"
"net/http"
"github.com/golang-jwt/jwt/v5"
)
func main() {
// We've already established our JWT middleware earlier - let's put it into action
// Set up our router and apply JWT middleware to our protected endpoints
myRouter := http.NewServeMux()
myRouter.Handle("/protected", JWTMiddleware(http.HandlerFunc(ProtectedEndpoint)))
// Run our server
fmt.Println("Serving on http://localhost:8080...")
http.ListenAndServe(":8080", myRouter)
}
func ProtectedEndpoint(w http.ResponseWriter, r *http.Request) {
// This is your shielded stronghold; only the worthy (authenticated users) make it here.
fmt.Fprintln(w, "Congratulations! You've passed the JWT authentication gates!")
}
Role-Based Access Control with JWT Claims
Some treasures are meant for only the most esteemed visitors. That's where role-based access control (RBAC) enters the scene. We can use the claims within our JWT to grant varying levels of access to different users.
// You'll be adjusting JWTMiddleware to not only validate tokens but also check roles
func JWTMiddleware(next http.Handler) http.Handler {
return http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) {
// ... existing token validation ...
// Assert role claims for RBAC
if claims, ok := token.Claims.(*jwt.RegisteredClaims); ok && token.Valid {
userRole := claims["role"]
// Check for the required role to access the endpoint
if userRole != "admin" {
http.Error(w, "You must be an admin to access this", http.StatusForbidden)
return
}
} else {
http.Error(w, "Invalid token claims", http.StatusForbidden)
return
}
// Token and roles are valid; proceed to the protected handler
next.ServeHTTP(w, r)
})
}
By conjuring the role claim from within our token, we turn the key only for those with the 'admin' insignia. All others shall return from whence they came, met with a formidable 403 refusal.
And with a wave of our Go wand, our endpoints are now a citadel, accessible only to those with the right JWT keys. Our quest nears its end, but the adventure of fortifying Go apps never truly ceases. Go forth and secure, brave code warriors!
Error Handling and Best Practices
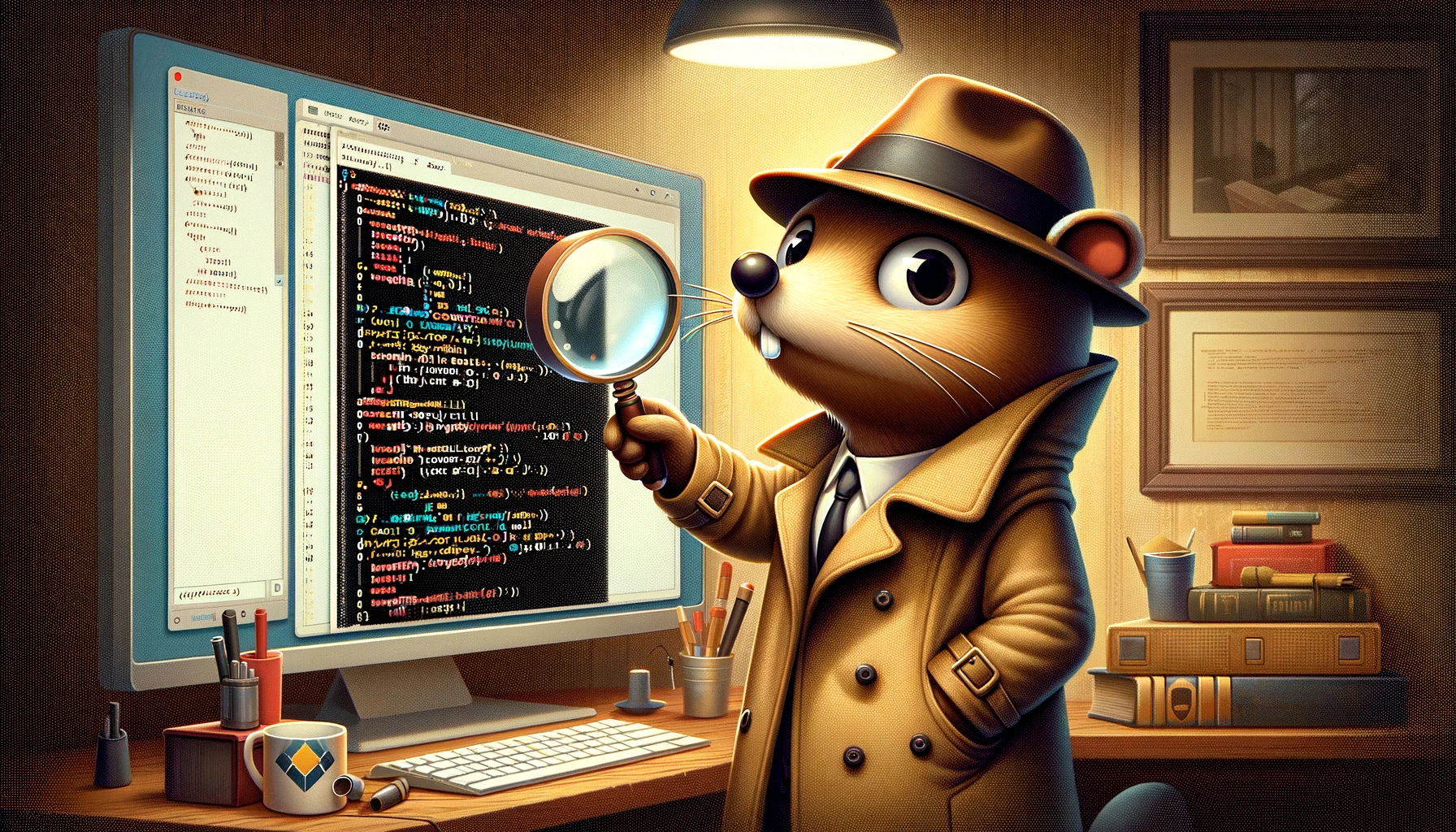
As we tread through the thickets of code, let's not forget to pave our path with the cobblestones of error handling and best practices. Nobody likes to trip over an unexpected `nil` pointer or be ambushed by a silent fail. Let's raise our lanterns high and light the way right!
Handling Common JWT Authentication Errors
No journey is without its perils, and in our JWT adventure, that often translates to handling authentication errors. A securely tight middleware is not just good for repelling invaders, but also for guiding lost travelers—errant tokens, in our case—back to the light.
Here's how one might spruce up our existing middleware for illumination:
package main
import (
"net/http"
"github.com/golang-jwt/jwt/v5"
)
// (Inside your middleware function)
// Parse the JWT string and store the result in `claims`.
token, err := jwt.ParseWithClaims(tokenStr, claims, keyFunc)
if err != nil {
var errResp string
switch e := err.(type) {
case *jwt.ValidationError: // something was wrong with the validation
switch e.Errors {
case jwt.ValidationErrorExpired:
errResp = "Your token has expired."
case jwt.ValidationErrorNotValidYet:
errResp = "Your token is not valid yet."
default:
errResp = "Your token could not be validated."
}
default:
errResp = "An internal error occurred."
}
http.Error(w, errResp, http.StatusUnauthorized)
return
}
// ... (token validation continues)
Giving clear feedback is as crucial as hanging a "Beware of the Dragon" sign outside a lair. It not only helps those interacting with your API but also aids in debugging during development.
Tips for Securely Storing and Transmitting Tokens
Now, a few pearls of wisdom for securely storing and transmitting those sacred JWTs:
- Never hard-code your secrets: Store them safely using environment variables or secret management tools.
- Use HTTPS: Unencrypted HTTP is like sending a raven without a cage; you never know who might snatch it mid-flight. Always encrypt communication with TLS/SSL.
- Consider token theft: If a token is stolen, it can be used by an attacker. Implement measures like refresh tokens and token revocation to mitigate this risk.
- Don't store sensitive information in your JWT: Tokens are not vaults. They can be decoded easily, so avoid placing any confidential data in them.
And there we go, worthy coders! With these bulwarks in place, our voyage through the JWT implementation is robust and vigilant. Remember that even the strongest fortifications require upkeep—stay informed on the latest security practices, and don't let those walls crumble!
Testing Your JWT Implementation
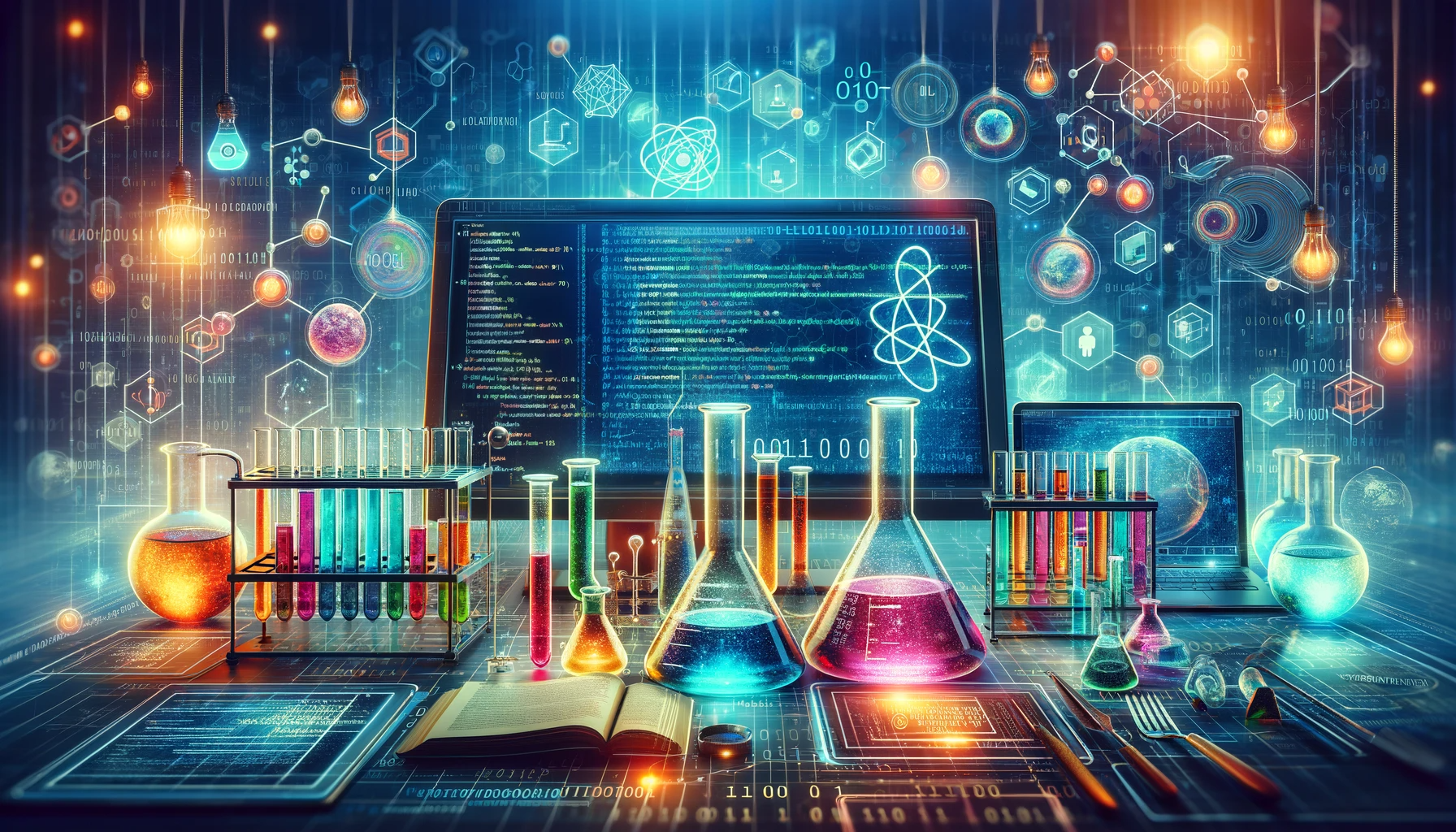
What good is a treasure chest without a sturdy lock? Just as a blacksmith tests their locks, we must test our JWT implementation to ensure it keeps our digital treasures safe. In this chapter, we'll delve into the craft of testing our Go application's JWT functionality.
Unit Testing the Token Generation and Validation
The cornerstone of reliable software is a solid suite of tests. Let our quest begin with unit tests for token generation and validation.
package main
import (
"testing"
"time"
"github.com/golang-jwt/jwt/v5"
"github.com/stretchr/testify/assert"
)
func TestTokenGenerationAndValidation(t *testing.T) {
jwtKey := "testKey"
claims := &jwt.RegisteredClaims{
ExpiresAt: jwt.NewNumericDate(time.Now().Add(15 * time.Minute)),
Issuer: "testIssuer",
}
token := jwt.NewWithClaims(jwt.SigningMethodHS256, claims)
tokenString, err := token.SignedString([]byte(jwtKey))
assert.NoError(t, err, "Token generation should not throw an error")
validatedToken, err := jwt.ParseWithClaims(tokenString, &jwt.RegisteredClaims{}, func(token *jwt.Token) (interface{}, error) {
return []byte(jwtKey), nil
})
assert.NoError(t, err, "Token validation should not throw an error")
assert.True(t, validatedToken.Valid, "The token should be valid")
}
With our trusty `assert` library, we ensure our token forging and verifying mechanisms work seamlessly. Plunge your swords into this battleground of tests to emerge victorious!
Integration Testing with Protected Endpoints
Now, let’s broaden our minds and our tests to include the guarding of our protected endpoints. The drawbridge must lift seamlessly upon token presentation!
func TestProtectedEndpoint(t *testing.T) {
// We generate a token as an authenticated user would receive
token := generateTestJWT("testKey", "testIssuer")
// Mock a request to our protected endpoint with the token in the header
req, _ := http.NewRequest("GET", "/protected", nil)
req.Header.Set("Authorization", "Bearer "+token)
// Record the response
rr := httptest.NewRecorder()
handler := http.HandlerFunc(ProtectedEndpoint)
// Apply our middleware to the handler and serve the request
JWTMiddleware(handler).ServeHTTP(rr, req)
// Check for the expected status code.
assert.Equal(t, http.StatusOK, rr.Code, "Handler returned wrong status code")
}
With the grand tapestry of HTTP testing, we simulate the clash of requests and our authentication barriers. We wield `httptest` and the assert library to verify that only the righteous may pass.
Best Practices for Test Coverage and Maintenance
A sturdy defense requires vigilance: endeavor to cover not only the success paths but also the various states of dismay—expired tokens, invalid signatures, and malformed tokens.
- Cover edge cases: Aim for thoroughness; forge tests for all possible authentication scenarios and token states.
- Continuous integration: Integrate your tests with your CI pipeline to ardently guard your fort against regression.
- Keep tests up-to-date: As the JWT standard and Go packages evolve, so too should your tests—keep them fresh and relevant.
Only through rigour and routine can one's castle stand unyielding. Equip your JWT implementation with a robust testing framework, and let it be known that your application is as impenetrable as they come!
Scaling and Maintenance
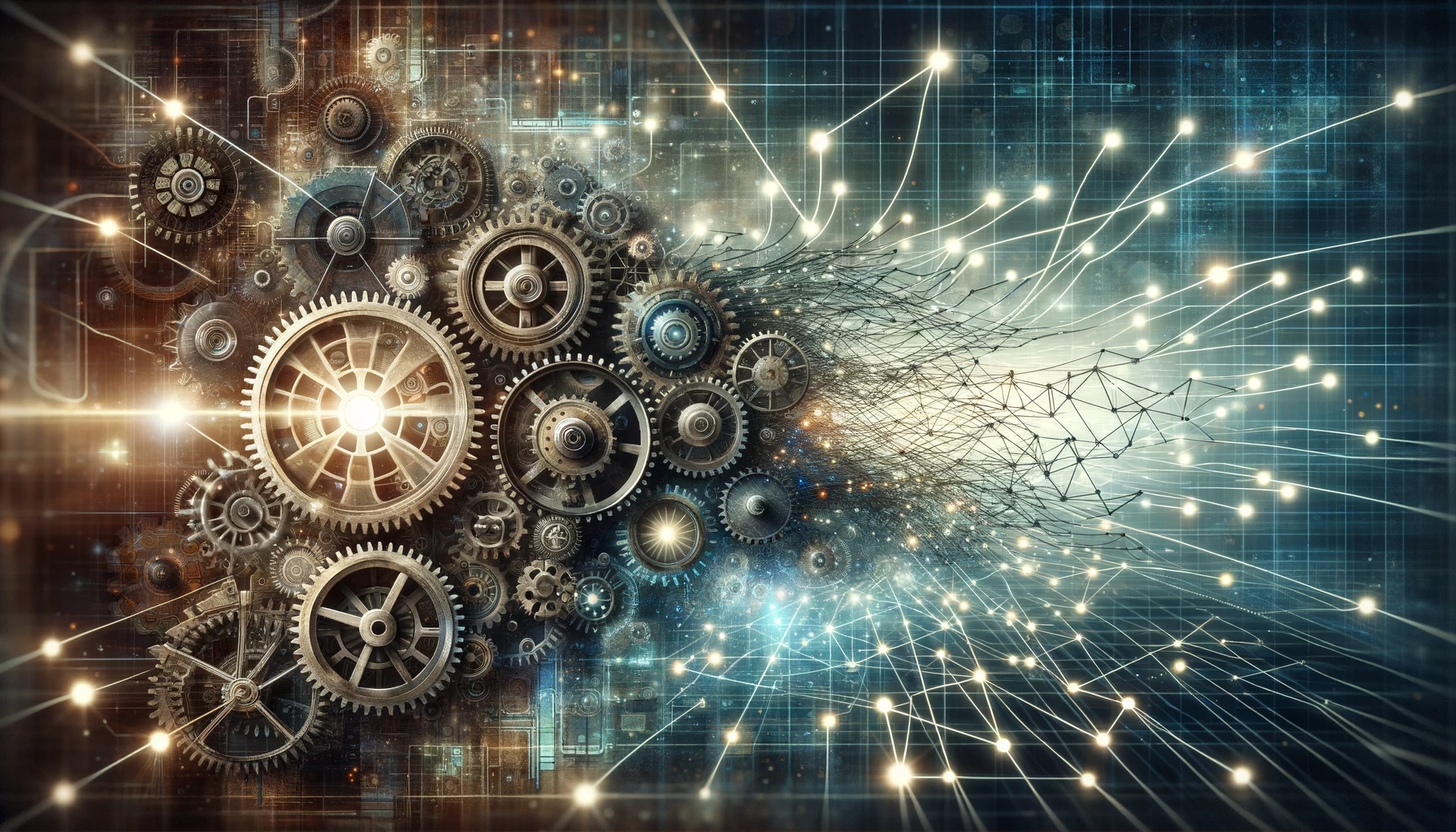
Maintaining a realm of code not only calls for the craftsmanship of a skilled blacksmith but also the foresight of a master strategist. As your application grows and battles tougher challenges, it is essential to prepare its authentication mechanisms to scale effectively.
Optimizing Performance for JWT Operations
To ensure the battlements hold strong as the hordes increase, optimizations in the JWT processes are key. Let's explore the ways to keep your JWT operations optimal:
package main
// Assume we have a global JWT secret manager
var jwtSecrets *SecretManager
// This function caches and retrieves JWT secrets efficiently
func GetSigningKey(token *jwt.Token) (interface{}, error) {
// Check if the signing method is as expected
if _, ok := token.Method.(*jwt.SigningMethodHMAC); !ok {
return nil, fmt.Errorf("unexpected signing method: %v", token.Header["alg"])
}
// Retrieve and return the secret from the manager
return jwtSecrets.Get(), nil
}
// For scaling, the cache can be connected to Redis, memcache, or a similar distributed system.
This Go snippet demonstrates the use of a hypothetical `SecretManager` which would cache JWT secrets to optimize performance and prepare for scalability.
Ensuring Reliability in Distributed Systems
As the number of loyal subjects to your app kingdom mounts, the need for JWT handling across distributed systems becomes inevitable. Let's take a glance at incorporating a JWT validation service:
// A JWT validation service running independently in your distributed system
func ValidateTokenService(tokenStr string) (*jwt.RegisteredClaims, error) {
// We're imagining a service that parses and validates a token, and returns claims if it's valid
token, err := jwt.ParseWithClaims(tokenStr, &jwt.RegisteredClaims{}, GetSigningKey)
if err != nil {
return nil, err
}
if claims, ok := token.Claims.(*jwt.RegisteredClaims); ok && token.Valid {
return claims, nil
} else {
return nil, jwt.NewValidationError("invalid token", jwt.ValidationErrorInvalid)
}
}
This code points the way for JWT validation to be offloaded to an independent service within your application's ecosystem, promoting better scalability and separation of concerns.
Best Practices for Scaling and Maintaining JWT Systems
And now, knights of the round table of Go, here be a list of the best practices to keep your JWT infrastructure stalwart:
- Centralize secret management: A strong secret management process is key to scaling. Consider services like HashiCorp's Vault or AWS Secrets Manager to manage secrets across services.
- Use load balancers: They will ensure the equal distribution of authentication requests across your services.
- Monitor and log: Keep an eye on your authentication mechanisms. Logging access and errors can prevent issues from turning into critical system failures.
- Regularly review and rotate keys: Regular updating of signing keys as a security best practice can help avoid long-term exploits.
With these tenets in place, your JWT fortress will be both mighty and wise, ever-ready to grow stronger with each day's new dawn. Till next we meet on the battlefield of bytes!
Conclusion
Our grand tour of safeguarding realms with JWTs in the world of Go applications draws to a close. We have traversed through the intricate weaving of tokensmithery, the strategic fortification of endpoints, and the rigorous training grounds of testing.
Let's recap the knowledge gained in our journey:
- We started by learning the arcane arts of Generating Tokens, employing the venerable
github.com/golang-jwt/jwt/v5
package in Go. - Next, we armored our endpoints for battle through Securing Endpoints with JWT, creating a bastion of safety for our users' requests.
- Our saga continued as we navigated the treacherous paths of Error Handling and Best Practices, etching wisdom into our code to prevent and respond to any missteps gracefully.
- No strategy is complete without rigorously Testing Your JWT Implementation, ensuring that every token generation and verification is a ceremony of solidity and trust.
- And finally, we prepared for the growth of our digital kingdom with Scaling and Maintenance practices that will stand the test of time and user demand.
As we stand atop our digital citadels, let us not forget that the realm of code is ever-evolving. Keep your libraries up to date, continue to forge new tests as you expand your empire, and never cease in your quest to learn and improve your craft.
May your tokens remain valid, your endpoints securely guarded, and your codebase robust against the ever-changing tides of user needs and security challenges. Thus we close the book on this chapter, but remember — the end of one quest is but the prelude to the next. Onward to further coding adventures!