Hey there, fellow developers! If you're anything like me, you're probably always on the hunt for the next cool thing in the world of programming. Well, today, I'm going to dive into something that's been buzzing in the developer community: Go, specifically creating APIs with the Gin framework. Now, before you roll your eyes thinking it's just another tech fad, let me assure you, it's not. Go is like that reliable friend who's always there to make your life easier, and Gin? It's the cherry on top.
For the uninitiated, Go, also affectionately known as Golang, is Google's brainchild - a language that combines simplicity, robust performance, and a touch of fun. Why Go for API development, you ask? Its simplicity and efficiency are like a breath of fresh air. And when you pair it with Gin, a high-performance micro-framework, you're signing up for an API development experience that's as smooth as a well-mixed gin and tonic.
In this post, I'm going to walk you through the ins and outs of creating an API in Go using Gin. Whether you're a seasoned pro in Go or just dipping your toes in, there's something for everyone. So, grab your favorite beverage (mine's a coffee, no gin before five!), and let's get started. By the end of this, you'll not only have a solid understanding of the Go-Gin combo but also a functional API that you can proudly show off. Let's code!
// A sneak peek into the simplicity of Go with Gin
package main
import "github.com/gin-gonic/gin"
func main() {
r := gin.Default()
r.GET("/ping", func(c *gin.Context) {
c.JSON(200, gin.H{
"message": "pong",
})
})
r.Run() // listen and serve on 0.0.0.0:8080
}
Section 1: Getting Started with Gin in Go
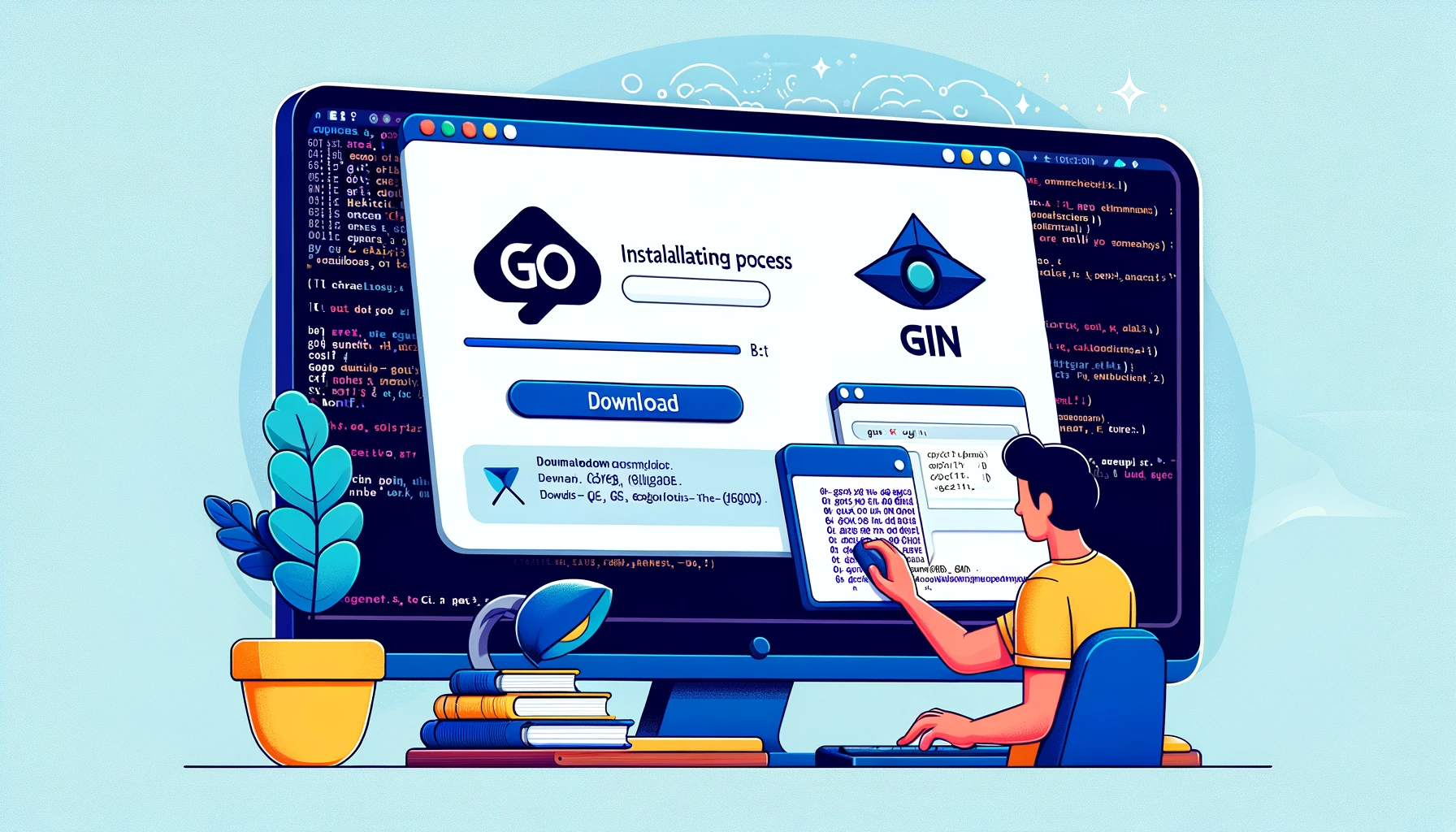
Setting up the Go environment
Before we jump into the Gin pool, let's get our Go environment ready. If you're new to Go, welcome to the club! Installing Go is like setting up a new gaming console; it's exciting and the first step to hours of fun. Head over to the official Go download page and grab the latest version. Once installed, let's set up your Go workspace. In the Go world, organization is key. Create a directory for your Go projects (mine's creatively named 'GoProjects'), and you're all set. Remember to set your GOPATH environment variable; it tells Go where to look for your code.
$ mkdir GoProjects
$ export GOPATH=$(pwd)/GoProjects
Installing and configuring Gin
Now for the star of the show - Gin. Since you prefer using Go modules (a wise choice for easy dependency management), let's initialize a new module. Create a new directory for our Gin project and initialize a Go module there. We'll call our project 'gin-api' because why not?
$ mkdir gin-api
$ cd gin-api
$ go mod init gin-api
With our module initialized, installing Gin is as easy as adding a new toy to your shopping cart. Run the following command to get Gin:
$ go get -u github.com/gin-gonic/gin
And voilà, you've just added Gin to your Go project! Our basic configuration is set. At this point, your 'gin-api' directory should have a 'go.mod' file that includes Gin as a dependency. This is Go's way of saying, "Got everything noted, boss!"
// Your go.mod file should look something like this
module gin-api
go 1.16
require github.com/gin-gonic/gin v1.7.2 // indirect
That's it for the setup! You're now armed with Go and Gin, ready to build something amazing. In the next section, we'll dive into creating your very first API endpoint with Gin. It's going to be a thrilling ride, so hold on to your keyboards!
Section 2: Building Your First API Endpoint with Gin
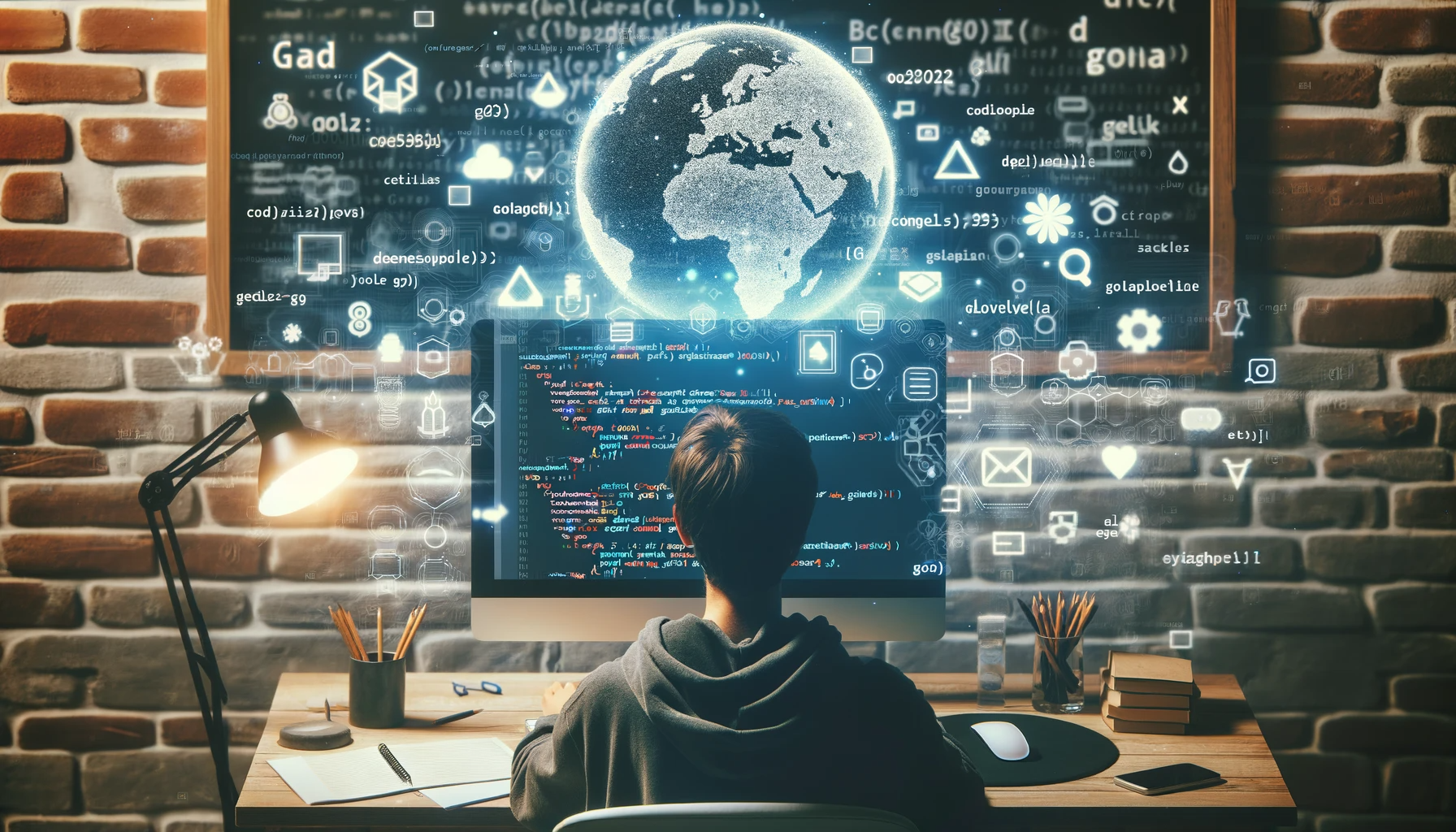
Understanding Routing in Gin
Now that we've got Go and Gin shaking hands, it's time to play matchmaker and create our first API endpoint. But first, let's talk routing. In the world of web servers, routing is like the GPS that directs requests to the correct destination. In Gin, it's a breeze. You define routes and associate them with handler functions that execute your business logic. Think of it as telling your app, "Hey, when someone asks for '/hello', show them how friendly we are."
func main() {
r := gin.Default()
r.GET("/hello", func(c *gin.Context) {
c.String(http.StatusOK, "Hello, world!")
})
r.Run() // listen on 0.0.0.0:8080
}
Here, we've defined a simple route '/hello' that responds with a warm "Hello, world!". It's the 'Hello World' of the API world.
Creating a simple “Hello World” API endpoint
Let's get our hands dirty by creating this 'Hello World' API endpoint. In your 'gin-api' directory, create a file named 'main.go'. This is where our Gin magic begins. Write the following code to set up a basic Gin router and define a GET route. When someone visits '/hello', our API will greet them like an old friend.
// main.go
package main
import (
"net/http"
"github.com/gin-gonic/gin"
)
func main() {
router := gin.Default()
router.GET("/hello", func(c *gin.Context) {
c.String(http.StatusOK, "Hello, world!")
})
router.Run() // listen and serve on 0.0.0.0:8080
}
Run your Go application with go run main.go
, and navigate to http://localhost:8080/hello
in your browser or use a tool like Postman to send a GET request. You should be greeted with a friendly "Hello, world!" message.
Testing the API endpoint
No API is complete without a test drive. Testing in Go is like checking your car before a long trip – essential for a smooth journey. Create a new file named 'main_test.go' in the same directory. We'll write a simple test to ensure our '/hello' endpoint is up and running.
// main_test.go
package main
import (
"net/http"
"net/http/httptest"
"testing"
"github.com/gin-gonic/gin"
"github.com/stretchr/testify/assert"
)
func TestHelloWorldEndpoint(t *testing.T) {
router := gin.Default()
router.GET("/hello", func(c *gin.Context) {
c.String(http.StatusOK, "Hello, world!")
})
w := httptest.NewRecorder()
req, _ := http.NewRequest("GET", "/hello", nil)
router.ServeHTTP(w, req)
assert.Equal(t, http.StatusOK, w.Code)
assert.Equal(t, "Hello, world!", w.Body.String())
}
Run your tests with go test
. If everything's set up right, you should see a passing test, signaling that your API endpoint is responding as expected. Congratulations, you've just created and tested your first API endpoint with Gin in Go! Give yourself a pat on the back, or better yet, a coffee break – you've earned it!
Section 3: Implementing CRUD Operations
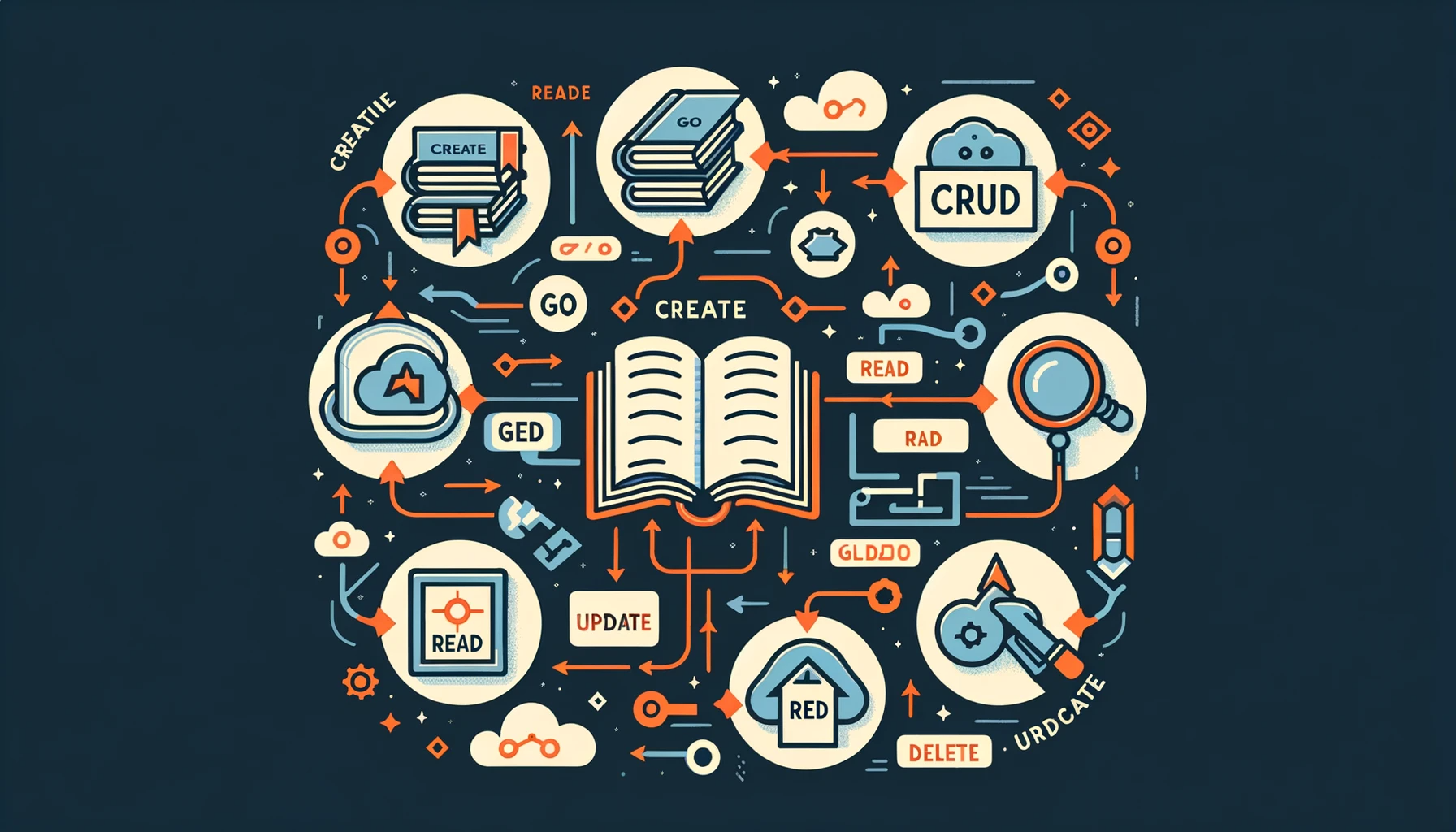
Setting up a mock database
Every good API needs data to play with, but let's not get bogged down with complex database setups just yet. We'll use a mock database, a fancy term for "let's pretend." Imagine a little in-memory structure to store our data. It's like playing house for APIs. Below is a simple mock structure to simulate a database with some basic data.
// Our mock database
var mockDB = map[string]string{
"1": "Gin for Dummies",
"2": "Mastering Go",
}
// Book represents our data model
type Book struct {
ID string `json:"id"`
Title string `json:"title"`
}
We're creating a simple map to store book titles with their IDs and defining a 'Book' struct to represent our data model. Simple and effective!
Building CRUD endpoints
CRUD stands for Create, Read, Update, and Delete - the four basic operations of persistent storage. Think of it as the life cycle of data. Now, let's implement these operations in our API.
Creating a Create endpoint
First up, the 'Create' operation. We want to add new books to our mock database. Here's how we do it:
router.POST("/book", func(c *gin.Context) {
var newBook Book
if err := c.BindJSON(&newBook); err != nil {
return
}
mockDB[newBook.ID] = newBook.Title
c.IndentedJSON(http.StatusCreated, newBook)
})
This route listens for POST requests and adds a new book to our mock database. We use BindJSON
to bind the request body to our Book struct.
Developing Read, Update, and Delete endpoints
Next, let's implement the Read, Update, and Delete operations. These will allow us to fetch, modify, and remove data from our mock database, respectively.
// Read endpoint
router.GET("/book/:id", func(c *gin.Context) {
id := c.Param("id")
if title, ok := mockDB[id]; ok {
c.IndentedJSON(http.StatusOK, Book{ID: id, Title: title})
} else {
c.IndentedJSON(http.StatusNotFound, gin.H{"message": "book not found"})
}
})
// Update endpoint
router.PUT("/book/:id", func(c *gin.Context) {
id := c.Param("id")
var updatedBook Book
if err := c.BindJSON(&updatedBook); err != nil {
return
}
mockDB[id] = updatedBook.Title
c.IndentedJSON(http.StatusOK, updatedBook)
})
// Delete endpoint
router.DELETE("/book/:id", func(c *gin.Context) {
id := c.Param("id")
if _, ok := mockDB[id]; ok {
delete(mockDB, id)
c.IndentedJSON(http.StatusOK, gin.H{"message": "book deleted"})
} else {
c.IndentedJSON(http.StatusNotFound, gin.H{"message": "book not found"})
}
})
With these routes, we can now read, update, and delete books in our mock database. It's like having your own little library, minus the late fees.
That's it for CRUD operations! You've just built the backbone of most web applications. Feel like a pro yet? You should! In the next section, we'll dive into Middleware and error handling, adding another layer of sophistication to our API. Stay tuned!
Section 4: Middleware and Error Handling
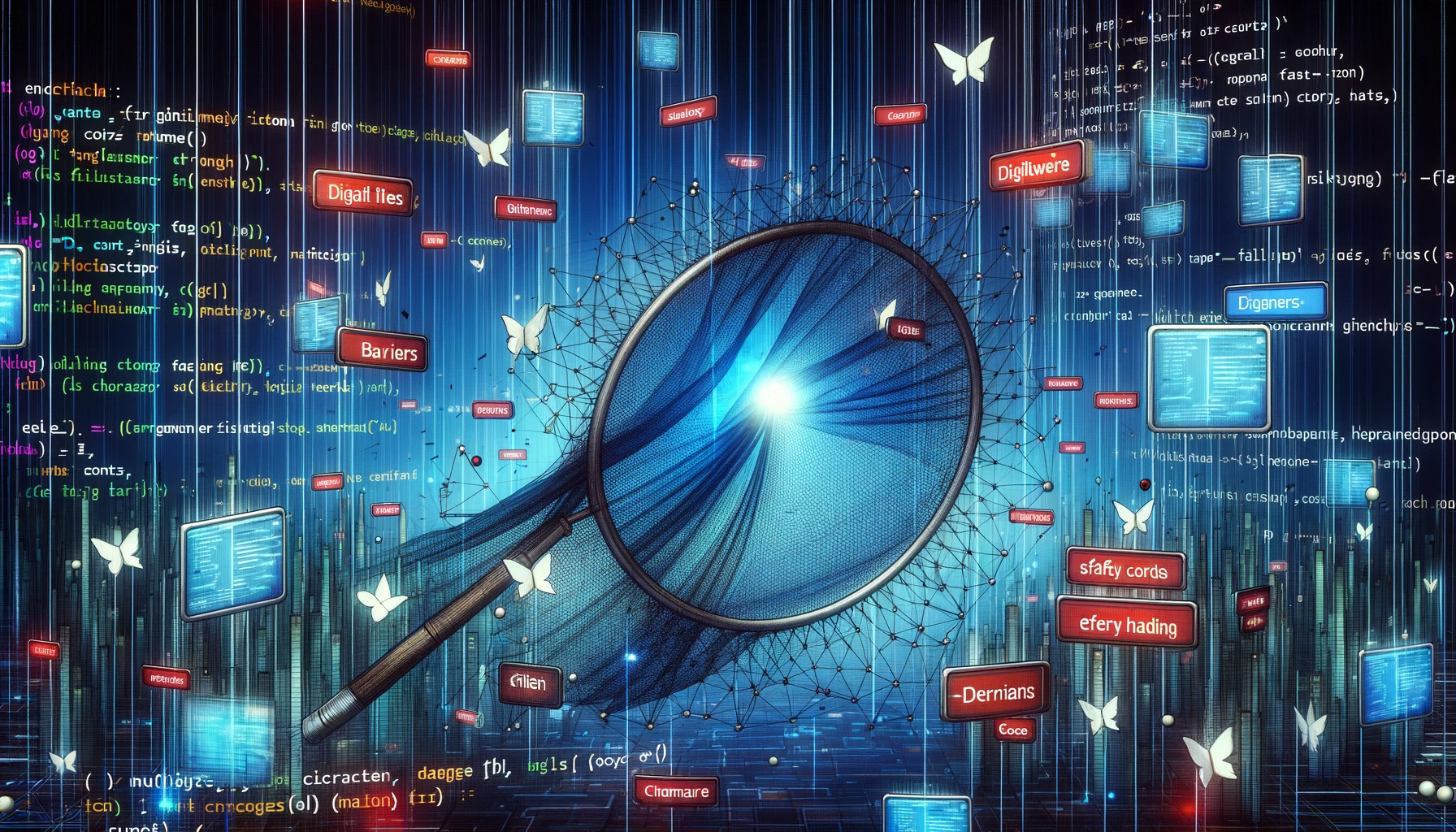
Understanding Middleware in Gin
Think of Middleware in Gin like the bouncers at a club. They check your ID, set the rules, and generally keep the peace. In the API world, Middleware helps manage HTTP requests and responses, handling things like logging, authentication, and more. It's like a Swiss Army knife for your routes, adding extra functionality before or after your main handlers run.
Middleware can be global, affecting all routes, or specific to certain routes. Gin's default logger and recovery (panic-recovery) middleware are perfect examples of global middleware, handling logging and preventing crashes for all routes.
Implementing custom Middleware
Now, let's roll up our sleeves and implement our own custom Middleware. We'll create a logging Middleware to keep an eye on how our API is being used. It's like having a little spy in your code, but in a good way.
Creating a logging Middleware
Our logging Middleware will log the time taken to process each request. This is incredibly useful for monitoring and optimizing API performance.
func LoggerMiddleware() gin.HandlerFunc {
return func(c *gin.Context) {
t := time.Now()
// Process request
c.Next()
// Log latency
latency := time.Since(t)
log.Printf("Handled request in %v", latency)
}
}
To use this Middleware, just add it to your router:
router := gin.Default()
router.Use(LoggerMiddleware())
Every request to your API will now be logged with its processing time. It's like keeping a diary for your API, but without the teenage angst.
Setting up error handling Middleware
Next up, error handling. Let's face it, errors are like uninvited party crashers. Our Middleware will gracefully handle these crashes and keep the API running smoothly.
func ErrorHandlingMiddleware() gin.HandlerFunc {
return func(c *gin.Context) {
defer func() {
if err := recover(); err != nil {
log.Printf("Recovered from error: %v", err)
c.AbortWithStatus(http.StatusInternalServerError)
}
}()
c.Next()
}
}
Add this Middleware to your router to protect your API from unexpected errors:
router.Use(ErrorHandlingMiddleware())
This Middleware catches any panics, logs the error, and returns a 500 Internal Server Error response. It's like having an airbag in your car; you hope you never need it, but you're glad it's there.
With Middleware in place, your API is now smarter, safer, and more sophisticated. It's like giving your API a PhD in Computer Science. In the next section, we'll look at testing and optimizing your API, putting the final touches on our Go-Gin masterpiece. Stay tuned!
Section 5: Testing and Optimizing Your API
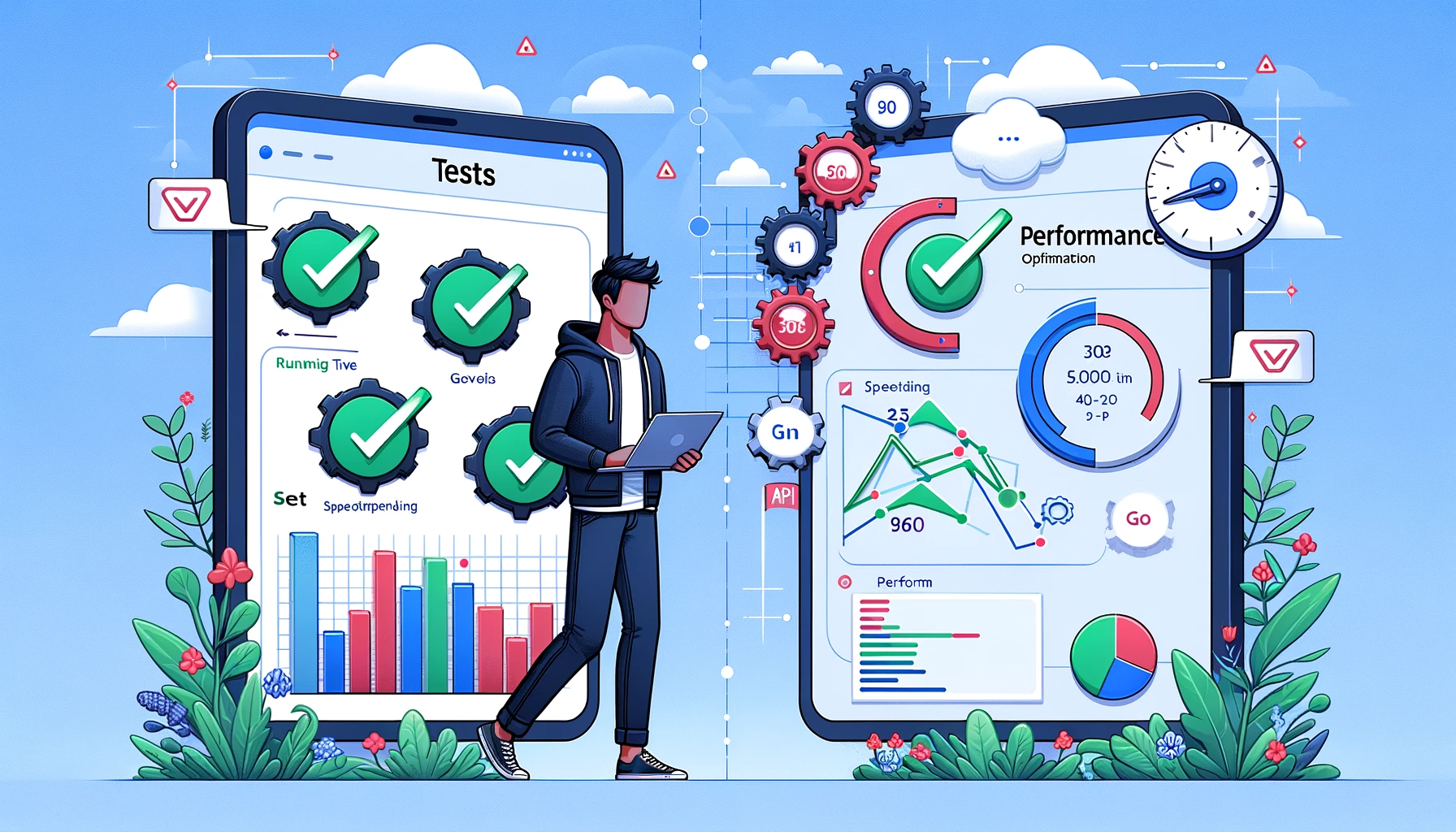
Writing tests for your Gin API
Testing in software development is like eating your vegetables – it might not always be the most exciting part of the meal, but it's essential for good health. In Go, thanks to its powerful standard library, writing tests is straightforward. Let's write some tests for our Gin API to ensure everything is running smoothly.
Start by creating a new file named `book_test.go` in your project. We'll write a test for one of our CRUD operations, say, fetching a book. Remember the mock database we set up earlier? We're going to use it here.
// book_test.go
package main
import (
"net/http"
"net/http/httptest"
"testing"
"github.com/gin-gonic/gin"
"github.com/stretchr/testify/assert"
)
func TestGetBook(t *testing.T) {
// Set up the router
router := gin.Default()
setupRouter(router)
// Perform a GET request with that handler.
w := httptest.NewRecorder()
req, _ := http.NewRequest("GET", "/book/1", nil)
router.ServeHTTP(w, req)
// Assert we got the correct response.
assert.Equal(t, http.StatusOK, w.Code)
assert.Contains(t, w.Body.String(), "Gin for Dummies")
}
Run your tests using the `go test` command. If all goes well, you should see a green light, indicating your API is in good health.
Tips for optimizing Gin API performance
Optimization is like tuning a car; you want it to run as efficiently as possible. When it comes to Go and Gin, there are several ways to boost performance.
First, let's talk about Go best practices. Go is designed for concurrency. Make use of Go routines for tasks that can be run in parallel. This can dramatically improve the throughput of your API.
As for Gin-specific tips, be mindful of middleware. While they're incredibly useful, they also add overhead to every request. Use them judiciously, only when necessary. Also, consider using Gin's built-in JSON validation for request binding, as it's optimized for performance.
router.POST("/book", func(c *gin.Context) {
var newBook Book
// Using Gin's built-in validation
if err := c.ShouldBindJSON(&newBook); err != nil {
c.JSON(http.StatusBadRequest, gin.H{"error": err.Error()})
return
}
// Rest of the code...
})
Lastly, keep your dependencies up to date. The Go and Gin communities are always working on performance improvements. Regularly updating your dependencies ensures you're getting the best out of these advancements.
There you have it, folks! You've just built, tested, and optimized an API using Go and Gin. You're now equipped to build robust, efficient APIs that are a joy to work with. Happy coding, and remember, the best way to learn is by doing. So, go forth and build amazing things!
Conclusion
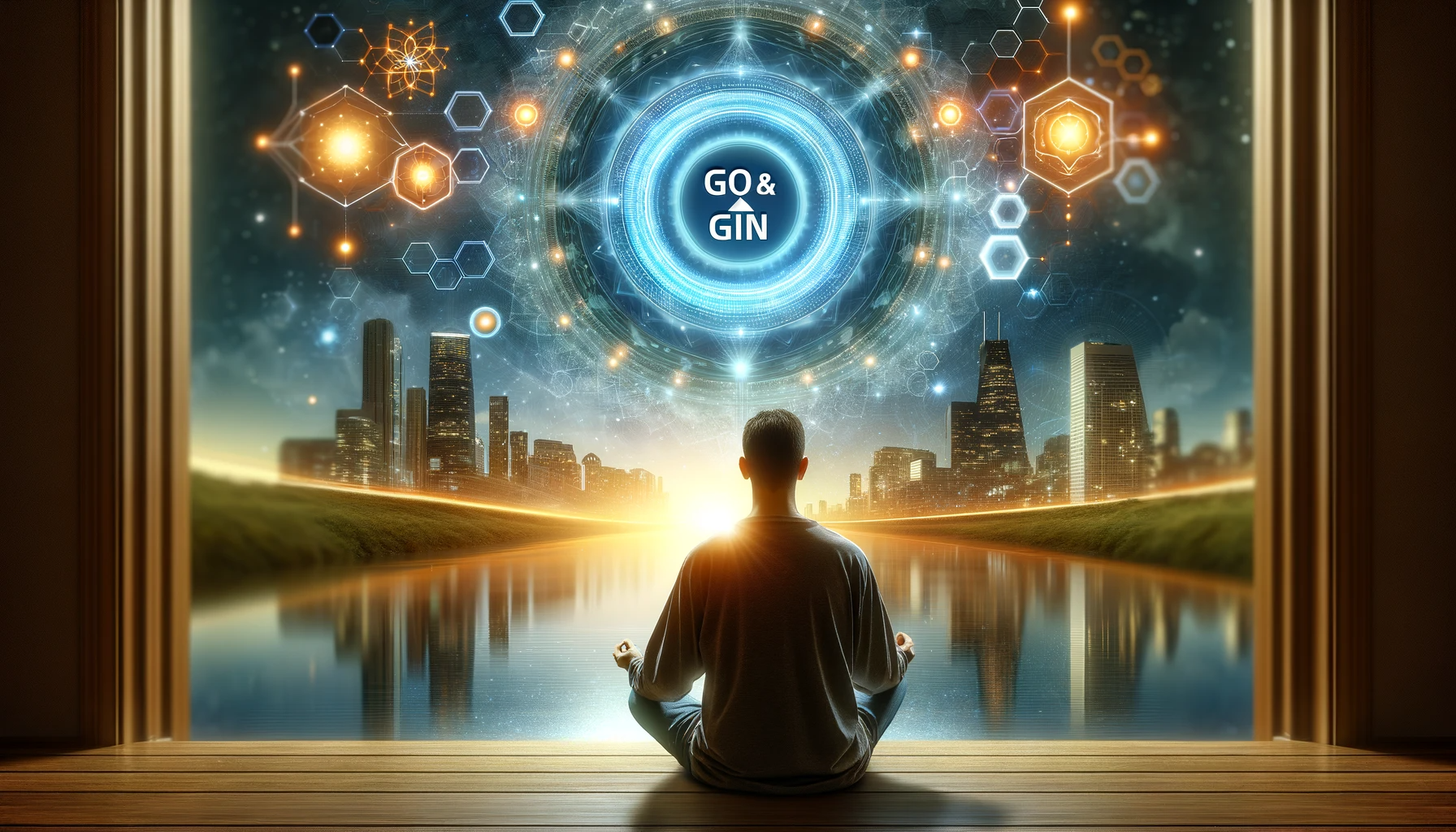
And there you have it, fellow coders! We've journeyed through the fascinating world of API development with Go and Gin, and I must say, it's been quite the adventure. From setting up our Go environment to mastering CRUD operations and even becoming savvy in middleware and error handling, we've covered some serious ground. We've seen how Go's simplicity and efficiency, combined with Gin's power and elegance, make for a formidable duo in API development.
But remember, this is just the beginning. The real fun starts when you apply these skills to your own projects. Go out there and build something amazing, something that makes you proud. Experiment with different features of Go and Gin, and don't be afraid to break things. That's how we learn, right? Plus, there's always the undo button.
If you're thirsty for more knowledge (because let's face it, learning is addictive), there are plenty of resources out there. The official Go documentation and the Gin GitHub repository are great places to start. And of course, the developer community is always there to help. Stack Overflow, Reddit, and Go forums are just a few clicks away.
Before I sign off, here's a parting tip: keep your Go code clean, simple, and idiomatic. It's not just about writing code that works; it's about writing code that speaks. After all, code is poetry, and every developer is a poet in their own right. Keep coding, keep learning, and most importantly, keep enjoying the process. Until next time, happy coding!